#include <StoreMap.h>
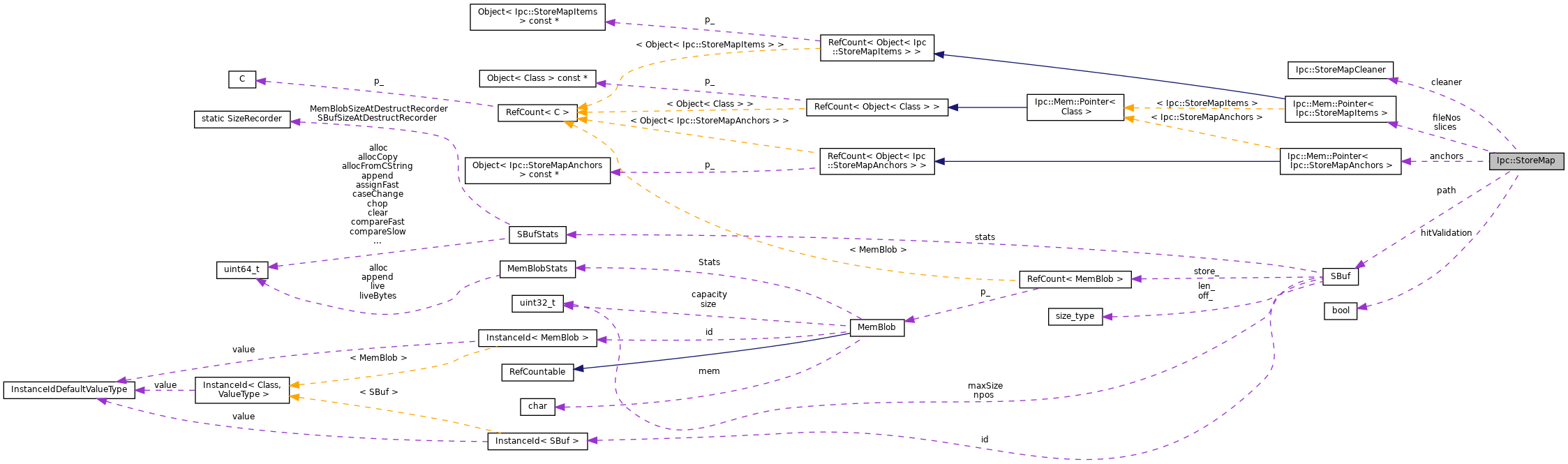
Classes | |
class | Owner |
aggregates anchor and slice owners for Init() caller convenience More... | |
Public Types | |
typedef StoreMapFileNos | FileNos |
typedef StoreMapAnchor | Anchor |
typedef StoreMapAnchors | Anchors |
typedef sfileno | AnchorId |
typedef StoreMapSlice | Slice |
typedef StoreMapSlices | Slices |
typedef StoreMapSliceId | SliceId |
typedef StoreMapUpdate | Update |
Public Member Functions | |
StoreMap (const SBuf &aPath) | |
sfileno | fileNoByKey (const cache_key *const key) const |
computes map entry anchor position for a given entry key More... | |
int | compareVersions (const sfileno oldFileno, time_t newVersion) const |
Anchor * | openForWriting (const cache_key *const key, sfileno &fileno) |
Anchor * | openForWritingAt (sfileno fileno, bool overwriteExisting=true) |
void | startAppending (const sfileno fileno) |
restrict opened for writing entry to appending operations; allow reads More... | |
void | closeForWriting (const sfileno fileno) |
successfully finish creating or updating the entry at fileno pos More... | |
void | switchWritingToReading (const sfileno fileno) |
stop writing (or updating) the locked entry and start reading it More... | |
void | forgetWritingEntry (const sfileno fileno) |
bool | openForUpdating (Update &update, sfileno fileNoHint) |
finds and locks the Update entry for an exclusive metadata update More... | |
void | closeForUpdating (Update &update) |
makes updated info available to others, unlocks, and cleans up More... | |
void | abortUpdating (Update &update) |
undoes partial update, unlocks, and cleans up More... | |
const Anchor * | peekAtReader (const sfileno fileno) const |
const Anchor * | peekAtWriter (const sfileno fileno) const |
const Anchor & | peekAtEntry (const sfileno fileno) const |
bool | freeEntry (const sfileno) |
void | freeEntryByKey (const cache_key *const key) |
bool | markedForDeletion (const cache_key *const) |
bool | hasReadableEntry (const cache_key *const) |
whether the index contains a valid readable entry with the given key More... | |
const Anchor * | openForReading (const cache_key *const key, sfileno &fileno) |
opens entry (identified by key) for reading, increments read level More... | |
const Anchor * | openForReadingAt (const sfileno, const cache_key *const) |
opens entry (identified by sfileno) for reading, increments read level More... | |
void | closeForReading (const sfileno fileno) |
closes open entry after reading, decrements read level More... | |
void | closeForReadingAndFreeIdle (const sfileno fileno) |
same as closeForReading() but also frees the entry if it is unlocked More... | |
const Anchor * | openOrCreateForReading (const cache_key *, sfileno &) |
openForReading() but creates a new entry if there is no old one More... | |
Slice & | writeableSlice (const AnchorId anchorId, const SliceId sliceId) |
writeable slice within an entry chain created by openForWriting() More... | |
const Slice & | readableSlice (const AnchorId anchorId, const SliceId sliceId) const |
readable slice within an entry chain opened by openForReading() More... | |
Anchor & | writeableEntry (const AnchorId anchorId) |
writeable anchor for the entry created by openForWriting() More... | |
const Anchor & | readableEntry (const AnchorId anchorId) const |
readable anchor for the entry created by openForReading() More... | |
void | prepFreeSlice (const SliceId sliceId) |
prepare a chain-unaffiliated slice for being added to an entry chain More... | |
SliceId | sliceContaining (const sfileno fileno, const uint64_t nth) const |
void | abortWriting (const sfileno fileno) |
stop writing the entry, freeing its slot for others to use if possible More... | |
bool | purgeOne () |
either finds and frees an entry with at least 1 slice or returns false More... | |
bool | validateHit (const sfileno) |
void | disableHitValidation () |
void | importSlice (const SliceId sliceId, const Slice &slice) |
copies slice to its designated position More... | |
bool | validEntry (const int n) const |
whether n is a valid slice coordinate More... | |
bool | validSlice (const int n) const |
whether n is a valid slice coordinate More... | |
int | entryCount () const |
number of writeable and readable entries More... | |
int | entryLimit () const |
maximum entryCount() possible More... | |
int | sliceLimit () const |
maximum number of slices possible More... | |
void | updateStats (ReadWriteLockStats &stats) const |
adds approximate current stats to the supplied ones More... | |
Static Public Member Functions | |
static Owner * | Init (const SBuf &path, const int slotLimit) |
initialize shared memory More... | |
Public Attributes | |
StoreMapCleaner * | cleaner |
notified before a readable entry is freed More... | |
Protected Attributes | |
const SBuf | path |
cache_dir path or similar cache name; for logging More... | |
Mem::Pointer< StoreMapFileNos > | fileNos |
entry inodes (starting blocks) More... | |
Mem::Pointer< StoreMapAnchors > | anchors |
entry inodes (starting blocks) More... | |
Mem::Pointer< StoreMapSlices > | slices |
chained entry pieces positions More... | |
Private Types | |
typedef std::function< bool(const sfileno name)> | NameFilter |
Private Member Functions | |
sfileno | nameByKey (const cache_key *const key) const |
computes entry name (i.e., key hash) for a given entry key More... | |
sfileno | fileNoByName (const sfileno name) const |
computes anchor position for a given entry name More... | |
void | relocate (const sfileno name, const sfileno fileno) |
map name to fileNo More... | |
Anchor & | anchorAt (const sfileno fileno) |
const Anchor & | anchorAt (const sfileno fileno) const |
Anchor & | anchorByKey (const cache_key *const key) |
Slice & | sliceAt (const SliceId sliceId) |
const Slice & | sliceAt (const SliceId sliceId) const |
Anchor * | openForReading (Slice &s) |
bool | openKeyless (Update::Edition &edition) |
void | closeForUpdateFinal (Update &update) |
bool | visitVictims (const NameFilter filter) |
void | freeChain (const sfileno fileno, Anchor &inode, const bool keepLock) |
unconditionally frees an already locked chain of slots, unlocking if needed More... | |
void | freeChainAt (SliceId sliceId, const SliceId splicingPoint) |
unconditionally frees an already locked chain of slots; no anchor maintenance More... | |
Private Attributes | |
bool | hitValidation |
whether paranoid_hit_validation should be performed More... | |
Detailed Description
Manages shared Store index (e.g., locking/unlocking/freeing entries) using StoreMapFileNos indexed by hashed entry keys (a.k.a. entry names), StoreMapAnchors indexed by fileno, and StoreMapSlices indexed by slice ID.
Definition at line 218 of file StoreMap.h.
Member Typedef Documentation
◆ Anchor
typedef StoreMapAnchor Ipc::StoreMap::Anchor |
Definition at line 222 of file StoreMap.h.
◆ AnchorId
typedef sfileno Ipc::StoreMap::AnchorId |
Definition at line 224 of file StoreMap.h.
◆ Anchors
Definition at line 223 of file StoreMap.h.
◆ FileNos
Definition at line 221 of file StoreMap.h.
◆ NameFilter
|
private |
Definition at line 386 of file StoreMap.h.
◆ Slice
typedef StoreMapSlice Ipc::StoreMap::Slice |
Definition at line 225 of file StoreMap.h.
◆ SliceId
Definition at line 227 of file StoreMap.h.
◆ Slices
typedef StoreMapSlices Ipc::StoreMap::Slices |
Definition at line 226 of file StoreMap.h.
◆ Update
typedef StoreMapUpdate Ipc::StoreMap::Update |
Definition at line 228 of file StoreMap.h.
Constructor & Destructor Documentation
◆ StoreMap()
Ipc::StoreMap::StoreMap | ( | const SBuf & | aPath | ) |
Definition at line 54 of file StoreMap.cc.
References anchors, assert, debugs, entryLimit(), fileNos, path, sliceLimit(), and slices.
Member Function Documentation
◆ abortUpdating()
void Ipc::StoreMap::abortUpdating | ( | Update & | update | ) |
Definition at line 268 of file StoreMap.cc.
References Ipc::StoreMapUpdate::Edition::anchor, Ipc::AssertFlagIsSet(), debugs, Ipc::StoreMapUpdate::Edition::fileNo, Ipc::StoreMapUpdate::fresh, Ipc::StoreMapAnchor::lock, Ipc::StoreMapUpdate::stale, Ipc::ReadWriteLock::unlockHeaders(), and Ipc::ReadWriteLock::updating.
Referenced by MemStore::updateHeaders().
◆ abortWriting()
void Ipc::StoreMap::abortWriting | ( | const sfileno | fileno | ) |
Definition at line 251 of file StoreMap.cc.
References Ipc::ReadWriteLock::appending, assert, debugs, Ipc::StoreMapAnchor::lock, Ipc::ReadWriteLock::stopAppendingAndRestoreExclusive(), Ipc::ReadWriteLock::unlockExclusive(), Ipc::StoreMapAnchor::waitingToBeFreed, Ipc::StoreMapAnchor::writerHalted, and Ipc::StoreMapAnchor::writing().
Referenced by MemStore::disconnect().
◆ anchorAt() [1/2]
|
private |
◆ anchorAt() [2/2]
|
private |
Definition at line 877 of file StoreMap.cc.
References anchorAt().
◆ anchorByKey()
|
private |
Definition at line 918 of file StoreMap.cc.
◆ closeForReading()
void Ipc::StoreMap::closeForReading | ( | const sfileno | fileno | ) |
Definition at line 496 of file StoreMap.cc.
References assert, debugs, Ipc::StoreMapAnchor::lock, Ipc::StoreMapAnchor::reading(), and Ipc::ReadWriteLock::unlockShared().
Referenced by MemStore::disconnect().
◆ closeForReadingAndFreeIdle()
void Ipc::StoreMap::closeForReadingAndFreeIdle | ( | const sfileno | fileno | ) |
Definition at line 505 of file StoreMap.cc.
References assert, and debugs.
Referenced by Transients::disconnect(), and Transients::get().
◆ closeForUpdateFinal()
|
private |
◆ closeForUpdating()
void Ipc::StoreMap::closeForUpdating | ( | Update & | update | ) |
Definition at line 604 of file StoreMap.cc.
References Ipc::StoreMapUpdate::Edition::anchor, Ipc::AssertFlagIsSet(), debugs, Ipc::StoreMapUpdate::entry, Ipc::StoreMapUpdate::Edition::fileNo, Ipc::StoreMapUpdate::fresh, Ipc::StoreMapAnchor::lock, Must, Ipc::StoreMapUpdate::Edition::name, Ipc::StoreMapSlice::next, Ipc::StoreMapSlice::size, Ipc::StoreMapAnchor::splicingPoint, Ipc::StoreMapUpdate::Edition::splicingPoint, Ipc::StoreMapUpdate::stale, Ipc::StoreMapAnchor::start, Ipc::ReadWriteLock::switchExclusiveToShared(), Ipc::ReadWriteLock::unlockHeaders(), Ipc::ReadWriteLock::updating, and Ipc::StoreMapAnchor::waitingToBeFreed.
Referenced by MemStore::updateHeadersOrThrow().
◆ closeForWriting()
void Ipc::StoreMap::closeForWriting | ( | const sfileno | fileno | ) |
Definition at line 200 of file StoreMap.cc.
References assert, debugs, Ipc::StoreMapAnchor::lock, Ipc::ReadWriteLock::unlockExclusive(), and Ipc::StoreMapAnchor::writing().
Referenced by MemStore::completeWriting(), and Transients::disconnect().
◆ compareVersions()
Like strcmp(mapped, new), but for store entry versions/timestamps. Returns +2 if the mapped entry does not exist; -1/0/+1 otherwise. Comparison may be inaccurate unless the caller is a lock holder.
Definition at line 68 of file StoreMap.cc.
References Ipc::StoreMapAnchor::basics, Ipc::StoreMapAnchor::empty(), and Ipc::StoreMapAnchor::Basics::timestamp.
◆ disableHitValidation()
|
inline |
Definition at line 346 of file StoreMap.h.
References hitValidation.
Referenced by Transients::init().
◆ entryCount()
int Ipc::StoreMap::entryCount | ( | ) | const |
Definition at line 738 of file StoreMap.cc.
Referenced by MemStore::currentCount(), Transients::currentCount(), and MemStore::reserveSapForWriting().
◆ entryLimit()
int Ipc::StoreMap::entryLimit | ( | ) | const |
Definition at line 732 of file StoreMap.cc.
References min(), and SwapFilenMax.
Referenced by StoreMap(), MemStore::stat(), and Transients::stat().
◆ fileNoByKey()
Definition at line 911 of file StoreMap.cc.
Referenced by Transients::evictIfFound().
◆ fileNoByName()
Definition at line 893 of file StoreMap.cc.
◆ forgetWritingEntry()
void Ipc::StoreMap::forgetWritingEntry | ( | const sfileno | fileno | ) |
unlock and "forget" openForWriting entry, making it Empty again this call does not free entry slices so the caller has to do that
Definition at line 84 of file StoreMap.cc.
References assert, debugs, Ipc::StoreMapAnchor::lock, Ipc::StoreMapAnchor::rewind(), Ipc::ReadWriteLock::unlockExclusive(), and Ipc::StoreMapAnchor::writing().
◆ freeChain()
Definition at line 374 of file StoreMap.cc.
References debugs, Ipc::StoreMapAnchor::empty(), Ipc::StoreMapAnchor::lock, Ipc::StoreMapAnchor::rewind(), Ipc::StoreMapAnchor::splicingPoint, Ipc::StoreMapAnchor::start, and Ipc::ReadWriteLock::unlockExclusive().
◆ freeChainAt()
Definition at line 390 of file StoreMap.cc.
References Ipc::StoreMapSlice::clear(), debugs, and Ipc::StoreMapSlice::next.
◆ freeEntry()
bool Ipc::StoreMap::freeEntry | ( | const sfileno | fileno | ) |
free the entry if possible or mark it as waiting to be freed if not
- Returns
- whether the entry was neither empty nor marked
Definition at line 312 of file StoreMap.cc.
References debugs, Ipc::StoreMapAnchor::empty(), Ipc::StoreMapAnchor::lock, Ipc::ReadWriteLock::lockExclusive(), and Ipc::StoreMapAnchor::waitingToBeFreed.
Referenced by MemStore::evictCached(), Transients::evictCached(), Transients::evictIfFound(), and MemStore::get().
◆ freeEntryByKey()
void Ipc::StoreMap::freeEntryByKey | ( | const cache_key *const | key | ) |
free the entry if possible or mark it as waiting to be freed if not does nothing if we cannot check that the key matches the cached entry
Definition at line 330 of file StoreMap.cc.
References debugs, Ipc::StoreMapAnchor::lock, Ipc::ReadWriteLock::lockExclusive(), Ipc::ReadWriteLock::lockShared(), Ipc::StoreMapAnchor::sameKey(), storeKeyText(), Ipc::ReadWriteLock::unlockExclusive(), Ipc::ReadWriteLock::unlockShared(), and Ipc::StoreMapAnchor::waitingToBeFreed.
Referenced by MemStore::evictIfFound().
◆ hasReadableEntry()
bool Ipc::StoreMap::hasReadableEntry | ( | const cache_key * const | key | ) |
Definition at line 362 of file StoreMap.cc.
◆ importSlice()
Definition at line 721 of file StoreMap.cc.
References assert.
◆ Init()
|
static |
Definition at line 42 of file StoreMap.cc.
References Ipc::StoreMap::Owner::anchors, assert, SBuf::c_str(), debugs, Ipc::StoreMap::Owner::fileNos, min(), path, shm_new, sliceLimit(), Ipc::StoreMap::Owner::slices, StoreMapAnchorsId(), StoreMapFileNosId(), StoreMapSlicesId(), and SwapFilenMax.
Referenced by MemStoreRr::create(), and TransientsRr::create().
◆ markedForDeletion()
bool Ipc::StoreMap::markedForDeletion | ( | const cache_key * const | key | ) |
whether the entry with the given key exists and was marked as "waiting to be freed" some time ago
Definition at line 354 of file StoreMap.cc.
References Ipc::StoreMapAnchor::sameKey(), and Ipc::StoreMapAnchor::waitingToBeFreed.
Referenced by Transients::markedForDeletion().
◆ nameByKey()
Definition at line 883 of file StoreMap.cc.
◆ openForReading() [1/2]
const Ipc::StoreMap::Anchor * Ipc::StoreMap::openForReading | ( | const cache_key *const | key, |
sfileno & | fileno | ||
) |
Definition at line 439 of file StoreMap.cc.
References debugs, and storeKeyText().
Referenced by MemStore::anchorToCache(), MemStore::get(), and Transients::get().
◆ openForReading() [2/2]
◆ openForReadingAt()
const Ipc::StoreMap::Anchor * Ipc::StoreMap::openForReadingAt | ( | const sfileno | fileno, |
const cache_key * const | key | ||
) |
Definition at line 452 of file StoreMap.cc.
References Config, debugs, Ipc::StoreMapAnchor::empty(), Ipc::StoreMapAnchor::lock, Ipc::ReadWriteLock::lockShared(), SquidConfig::paranoid_hit_validation, Ipc::StoreMapAnchor::sameKey(), Ipc::ReadWriteLock::unlockShared(), and Ipc::StoreMapAnchor::waitingToBeFreed.
◆ openForUpdating()
Definition at line 522 of file StoreMap.cc.
References Ipc::StoreMapUpdate::Edition::anchor, debugs, Ipc::StoreMapUpdate::entry, Ipc::StoreMapUpdate::Edition::fileNo, Ipc::StoreMapUpdate::fresh, hash_link::key, Ipc::StoreMapAnchor::lock, Ipc::ReadWriteLock::lockHeaders(), Must, Ipc::StoreMapUpdate::Edition::name, Ipc::StoreMapAnchor::set(), Ipc::StoreMapUpdate::stale, storeKeyText(), and Ipc::StoreMapAnchor::writing().
Referenced by MemStore::updateHeaders().
◆ openForWriting()
Ipc::StoreMap::Anchor * Ipc::StoreMap::openForWriting | ( | const cache_key *const | key, |
sfileno & | fileno | ||
) |
finds, locks, and returns an anchor for an empty key position, erasing the old entry (if any)
Definition at line 140 of file StoreMap.cc.
References debugs, and storeKeyText().
Referenced by Transients::addWriterEntry(), and MemStore::startCaching().
◆ openForWritingAt()
Ipc::StoreMap::Anchor * Ipc::StoreMap::openForWritingAt | ( | sfileno | fileno, |
bool | overwriteExisting = true |
||
) |
locks and returns an anchor for the empty fileno position; if overwriteExisting is false and the position is not empty, returns nil
Definition at line 155 of file StoreMap.cc.
References assert, debugs, Ipc::StoreMapAnchor::empty(), Ipc::StoreMapAnchor::lock, Ipc::ReadWriteLock::lockExclusive(), Ipc::StoreMapAnchor::reading(), Ipc::StoreMapAnchor::splicingPoint, Ipc::StoreMapAnchor::start, Ipc::ReadWriteLock::unlockExclusive(), Ipc::StoreMapAnchor::waitingToBeFreed, and Ipc::StoreMapAnchor::writing().
◆ openKeyless()
|
private |
finds an anchor that is currently not associated with any entry key and locks it for writing so ensure exclusive access during updates
Definition at line 586 of file StoreMap.cc.
References Ipc::StoreMapUpdate::Edition::anchor, debugs, Ipc::StoreMapUpdate::Edition::fileNo, Must, and Ipc::StoreMapUpdate::Edition::name.
◆ openOrCreateForReading()
const Ipc::StoreMap::Anchor * Ipc::StoreMap::openOrCreateForReading | ( | const cache_key * | key, |
sfileno & | fileno | ||
) |
Definition at line 103 of file StoreMap.cc.
References assert, debugs, and storeKeyText().
Referenced by Transients::addReaderEntry().
◆ peekAtEntry()
const Ipc::StoreMap::Anchor & Ipc::StoreMap::peekAtEntry | ( | const sfileno | fileno | ) | const |
the caller must hold a lock on the entry
- Returns
- the corresponding Anchor
Definition at line 306 of file StoreMap.cc.
Referenced by Transients::readers().
◆ peekAtReader()
const Ipc::StoreMap::Anchor * Ipc::StoreMap::peekAtReader | ( | const sfileno | fileno | ) | const |
the caller must hold a lock on the entry
- Returns
- nullptr unless the slice is readable
Definition at line 286 of file StoreMap.cc.
References assert, Ipc::StoreMapAnchor::reading(), and Ipc::StoreMapAnchor::writing().
◆ peekAtWriter()
const Ipc::StoreMap::Anchor * Ipc::StoreMap::peekAtWriter | ( | const sfileno | fileno | ) | const |
the caller must hold a lock on the entry
- Returns
- nullptr unless the slice is writeable
Definition at line 296 of file StoreMap.cc.
References assert, Ipc::StoreMapAnchor::reading(), and Ipc::StoreMapAnchor::writing().
Referenced by Transients::hasWriter().
◆ prepFreeSlice()
void Ipc::StoreMap::prepFreeSlice | ( | const SliceId | sliceId | ) |
Definition at line 412 of file StoreMap.cc.
References assert.
Referenced by MemStore::reserveSapForWriting().
◆ purgeOne()
bool Ipc::StoreMap::purgeOne | ( | ) |
Definition at line 701 of file StoreMap.cc.
References debugs, Ipc::StoreMapAnchor::empty(), Ipc::StoreMapAnchor::lock, Ipc::ReadWriteLock::lockExclusive(), Ipc::StoreMapAnchor::start, and Ipc::ReadWriteLock::unlockExclusive().
Referenced by MemStore::reserveSapForWriting().
◆ readableEntry()
const Ipc::StoreMap::Anchor & Ipc::StoreMap::readableEntry | ( | const AnchorId | anchorId | ) | const |
Definition at line 244 of file StoreMap.cc.
References assert.
Referenced by Transients::status(), and MemStore::updateAnchored().
◆ readableSlice()
const Ipc::StoreMap::Slice & Ipc::StoreMap::readableSlice | ( | const AnchorId | anchorId, |
const SliceId | sliceId | ||
) | const |
Definition at line 229 of file StoreMap.cc.
References assert.
Referenced by MemStore::copyFromShm(), and MemStore::updateHeadersOrThrow().
◆ relocate()
Definition at line 904 of file StoreMap.cc.
◆ sliceAt() [1/2]
|
private |
◆ sliceAt() [2/2]
|
private |
Definition at line 931 of file StoreMap.cc.
References sliceAt().
◆ sliceContaining()
Ipc::StoreMap::SliceId Ipc::StoreMap::sliceContaining | ( | const sfileno | fileno, |
const uint64_t | nth | ||
) | const |
Returns the ID of the entry slice containing n-th byte or a negative ID if the entry does not store that many bytes (yet). Requires a read lock.
Definition at line 420 of file StoreMap.cc.
References debugs, Must, Ipc::StoreMapSlice::next, Ipc::StoreMapAnchor::reading(), Ipc::StoreMapSlice::size, and Ipc::StoreMapAnchor::start.
Referenced by MemStore::updateHeadersOrThrow().
◆ sliceLimit()
int Ipc::StoreMap::sliceLimit | ( | ) | const |
Definition at line 744 of file StoreMap.cc.
Referenced by StoreMap(), Init(), and MemStore::stat().
◆ startAppending()
void Ipc::StoreMap::startAppending | ( | const sfileno | fileno | ) |
Definition at line 191 of file StoreMap.cc.
References assert, debugs, Ipc::StoreMapAnchor::lock, Ipc::ReadWriteLock::startAppending(), and Ipc::StoreMapAnchor::writing().
Referenced by Transients::addWriterEntry(), and MemStore::startCaching().
◆ switchWritingToReading()
void Ipc::StoreMap::switchWritingToReading | ( | const sfileno | fileno | ) |
Definition at line 211 of file StoreMap.cc.
References assert, Ipc::StoreMapAnchor::complete(), debugs, Ipc::StoreMapAnchor::lock, Ipc::ReadWriteLock::switchExclusiveToShared(), and Ipc::StoreMapAnchor::writing().
Referenced by Transients::completeWriting().
◆ updateStats()
void Ipc::StoreMap::updateStats | ( | ReadWriteLockStats & | stats | ) | const |
◆ validateHit()
bool Ipc::StoreMap::validateHit | ( | const sfileno | fileno | ) |
validates locked hit metadata and calls freeEntry() for invalid entries
- Returns
- whether hit metadata is correct
Definition at line 800 of file StoreMap.cc.
References StatCounters::attempts, Config, DBG_IMPORTANT, debugs, ConservativeTimer::expired(), StatCounters::failures, StatCounters::hitValidation, SquidConfig::paranoid_hit_validation, StatCounters::refusalsDueToLocking, StatCounters::refusalsDueToTimeLimit, StatCounters::refusalsDueToZeroSize, statCounter, and storeKeyText().
◆ validEntry()
bool Ipc::StoreMap::validEntry | ( | const int | n | ) | const |
Definition at line 757 of file StoreMap.cc.
◆ validSlice()
bool Ipc::StoreMap::validSlice | ( | const int | n | ) | const |
Definition at line 763 of file StoreMap.cc.
◆ visitVictims()
|
private |
Visits entries until either
- the
visitor
returns true (indicating its satisfaction with the offer); - we give up finding a suitable entry because it already took "too long"; or
- we have offered all entries.
Definition at line 684 of file StoreMap.cc.
◆ writeableEntry()
Ipc::StoreMap::Anchor & Ipc::StoreMap::writeableEntry | ( | const AnchorId | anchorId | ) |
Definition at line 237 of file StoreMap.cc.
References assert.
Referenced by MemStore::copyToShm(), MemStore::nextAppendableSlice(), and Transients::status().
◆ writeableSlice()
Ipc::StoreMap::Slice & Ipc::StoreMap::writeableSlice | ( | const AnchorId | anchorId, |
const SliceId | sliceId | ||
) |
Definition at line 221 of file StoreMap.cc.
References assert.
Referenced by MemStore::nextAppendableSlice().
Member Data Documentation
◆ anchors
|
protected |
Definition at line 366 of file StoreMap.h.
Referenced by StoreMap(), and Ipc::StoreMap::Owner::~Owner().
◆ cleaner
StoreMapCleaner* Ipc::StoreMap::cleaner |
Definition at line 361 of file StoreMap.h.
Referenced by MemStore::init(), and Transients::init().
◆ fileNos
|
protected |
Definition at line 365 of file StoreMap.h.
Referenced by StoreMap(), and Ipc::StoreMap::Owner::~Owner().
◆ hitValidation
|
private |
Definition at line 393 of file StoreMap.h.
Referenced by disableHitValidation().
◆ path
|
protected |
Definition at line 364 of file StoreMap.h.
Referenced by StoreMap(), and Init().
◆ slices
|
protected |
Definition at line 367 of file StoreMap.h.
Referenced by StoreMap(), and Ipc::StoreMap::Owner::~Owner().
The documentation for this class was generated from the following files:
- src/ipc/StoreMap.h
- src/ipc/StoreMap.cc